PYTHON LIST DEEP COPY
INTRODUCTION
In deep copy operation, changes in original list does not have the impact on copied list and vice-versa.
There are three ways we can create deep copy in Python.
- List Slicing Operation
- Importing copy Module to use deepcopy() method
- copy() Method for Primitive Data Types
List Slicing Operation
# Deep Copy operation using Slicing operator[:] example 1
org_list = [400, 200, 600, 100, 150, 500]
print("\nElement of original list ->", org_list)
copy_list = org_list[:]
print("\nElement of copied list ->", copy_list)
# Removing the last element from the copied list
copy_list.remove(600)
print("\nElement of original list ->", org_list)
print("\nElement of copied list ->", copy_list)
# UPdating the first element of the original list
org_list[0] = 700
print("\nElement of original list ->", org_list)
print("\nElement of copied list ->", copy_list)
PYTHON LIST DEEP COPY : Output
Element of original list -> [400, 200, 600, 100, 150, 500]
Element of copied list -> [400, 200, 600, 100, 150, 500]
Element of original list -> [400, 200, 600, 100, 150, 500]
Element of copied list -> [400, 200, 100, 150, 500]
Element of original list -> [700, 200, 600, 100, 150, 500]
Element of copied list -> [400, 200, 100, 150, 500]
In the above example, we can see that we have removed the element 600 from copied list and update the 1st element of original list to 700. But the impact of change in one list didn’t affect the other list. So, this is the deep copy.
We will now check the string list as well.
# Deep Copy operation using Slicing operator[:] example 2
org_list = ['Python', 'Java', 'C++', 'C', 'PHP', 'XML']
print("\nElement of original list ->", org_list)
copy_list = org_list[:]
print("\nElement of copied list ->", copy_list)
# Removing the last element from the copied list
copy_list.remove('C++')
print("\nElement of original list ->", org_list)
print("\nElement of copied list ->", copy_list)
# UPdating the first element of the original list
org_list[1] = 'Word'
print("\nElement of original list ->", org_list)
print("\nElement of copied list ->", copy_list)
PYTHON LIST DEEP COPY : Output
Element of original list -> ['Python', 'Java', 'C++', 'C', 'PHP', 'XML']
Element of copied list -> ['Python', 'Java', 'C++', 'C', 'PHP', 'XML']
Element of original list -> ['Python', 'Java', 'C++', 'C', 'PHP', 'XML']
Element of copied list -> ['Python', 'Java', 'C', 'PHP', 'XML']
Element of original list -> ['Python', 'Word', 'C++', 'C', 'PHP', 'XML']
Element of copied list -> ['Python', 'Java', 'C', 'PHP', 'XML']
Now we can see the same impact in string list as well.
Importing copy Module to use deepcopy() method
Impact on Primitive Data Type
import copy
org_list = [400, 200, 600, 100, 150, 500]
print("\nElement of original list ->", org_list)
copy_list = copy.deepcopy(org_list)
print("\nElement of copied list ->", copy_list)
# Removing the last element from the copied list
copy_list.remove(600)
print("\nElement of original list ->", org_list)
print("\nElement of copied list ->", copy_list)
# UPdating the first element of the original list
org_list[0] = 700
print("\nElement of original list ->", org_list)
print("\nElement of copied list ->", copy_list)
PYTHON LIST DEEP COPY : Output
Element of original list -> [400, 200, 600, 100, 150, 500]
Element of copied list -> [400, 200, 600, 100, 150, 500]
Element of original list -> [400, 200, 600, 100, 150, 500]
Element of copied list -> [400, 200, 100, 150, 500]
Element of original list -> [700, 200, 600, 100, 150, 500]
Element of copied list -> [400, 200, 100, 150, 500]
In the above example, we have imported the copy module in our program. After using deepcopy() method we can check that both original list and copied list have different set of elements. So the impact of changes in one list is not visible in the other list.
# Deep Copy operation using deepcopy() method example 2
import copy
org_list = ['Python', 'Java', 'C++', 'C', 'PHP', 'XML']
print("\nElement of original list ->", org_list)
copy_list = copy.deepcopy(org_list)
print("\nElement of copied list ->", copy_list)
# Removing the last element from the copied list
copy_list.remove('C++')
print("\nElement of original list ->", org_list)
print("\nElement of copied list ->", copy_list)
# UPdating the first element of the original list
org_list[1] = 'Word'
print("\nElement of original list ->", org_list)
print("\nElement of copied list ->", copy_list)
PYTHON LIST DEEP COPY : Output
Element of original list -> ['Python', 'Java', 'C++', 'C', 'PHP', 'XML']
Element of copied list -> ['Python', 'Java', 'C++', 'C', 'PHP', 'XML']
Element of original list -> ['Python', 'Java', 'C++', 'C', 'PHP', 'XML']
Element of copied list -> ['Python', 'Java', 'C', 'PHP', 'XML']
Element of original list -> ['Python', 'Word', 'C++', 'C', 'PHP', 'XML']
Element of copied list -> ['Python', 'Java', 'C', 'PHP', 'XML']
For string list also the deepcopy() method has the same impact.
Impact on Complex Data Type
Now we will see the impact of deepcopy() on list with complex data type.
# Deep Copy operation using deepcopy() method example 3
import copy
int_list = [1, 2, 5]
str_list = ['Python', 'Java', 'C++']
org_list = [400, 200, 600, 100, 150, 500, int_list, str_list]
print("\nElement of original list ->", org_list)
copy_list = copy.deepcopy(org_list)
print("\nElement of copied list ->", copy_list)
# Removing the last element from the copied list
int_list.remove(5)
print("\nElement of original list ->", org_list)
print("\nElement of copied list ->", copy_list)
# UPdating the first element of the original list
str_list[2] = 'Word'
print("\nElement of original list ->", org_list)
print("\nElement of copied list ->", copy_list)
PYTHON LIST DEEP COPY : Output
Element of original list -> [400, 200, 600, 100, 150, 500, [1, 2, 5], ['Python', 'Java', 'C++']]
Element of copied list -> [400, 200, 600, 100, 150, 500, [1, 2, 5], ['Python', 'Java', 'C++']]
Element of original list -> [400, 200, 600, 100, 150, 500, [1, 2], ['Python', 'Java', 'C++']]
Element of copied list -> [400, 200, 600, 100, 150, 500, [1, 2, 5], ['Python', 'Java', 'C++']]
Element of original list -> [400, 200, 600, 100, 150, 500, [1, 2], ['Python', 'Java', 'Word']]
Element of copied list -> [400, 200, 600, 100, 150, 500, [1, 2, 5], ['Python', 'Java', 'C++']]
In the above example, we have used two smaller list int_list and str_list inside the original list. We have modified both the smaller list. But the impact is visible only in the original list. So the deep copy impact is still intact for list having complex data type as well.
copy() Method for Primitive Data Types
If we use list having primitive data type only, then copy() method will also work like deep copy.
Integer Data Type
# Deep Copy operation using copy() method for primitive data types example 1
import copy
org_list = [400, 200, 600, 100, 150, 500]
print("\nElement of original list ->", org_list)
copy_list = copy.deepcopy(org_list)
print("\nElement of copied list ->", copy_list)
# Removing the last element from the copied list
org_list.remove(600)
print("\nElement of original list ->", org_list)
print("\nElement of copied list ->", copy_list)
# UPdating the first element of the original list
copy_list[0] = 700
print("\nElement of original list ->", org_list)
print("\nElement of copied list ->", copy_list)
PYTHON LIST DEEP COPY : Output
Element of original list -> [400, 200, 600, 100, 150, 500]
Element of copied list -> [400, 200, 600, 100, 150, 500]
Element of original list -> [400, 200, 100, 150, 500]
Element of copied list -> [400, 200, 600, 100, 150, 500]
Element of original list -> [400, 200, 100, 150, 500]
Element of copied list -> [700, 200, 600, 100, 150, 500]
String Data Type
# Deep Copy operation using copy() method for primitive data types example 2
import copy
org_list = ['Python', 'Java', 'C++', 'C', 'PHP', 'XML']
print("\nElement of original list ->", org_list)
copy_list = copy.deepcopy(org_list)
print("\nElement of copied list ->", copy_list)
# Removing the last element from the copied list
org_list.remove('C++')
print("\nElement of original list ->", org_list)
print("\nElement of copied list ->", copy_list)
# UPdating the first element of the original list
copy_list[2] = 'Word'
print("\nElement of original list ->", org_list)
print("\nElement of copied list ->", copy_list)
PYTHON LIST DEEP COPY : Output
Element of original list -> ['Python', 'Java', 'C++', 'C', 'PHP', 'XML']
Element of copied list -> ['Python', 'Java', 'C++', 'C', 'PHP', 'XML']
Element of original list -> ['Python', 'Java', 'C', 'PHP', 'XML']
Element of copied list -> ['Python', 'Java', 'C++', 'C', 'PHP', 'XML']
Element of original list -> ['Python', 'Java', 'C', 'PHP', 'XML']
Element of copied list -> ['Python', 'Java', 'Word', 'C', 'PHP', 'XML']
Both the example mentioned above, the original list and copied list are not in sync. So by using the copy() method we can achieve the deep copy feature if the elements in the list are of primitive data type.
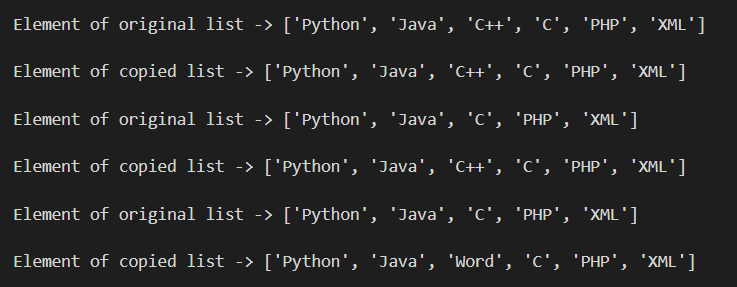
RELATED TOPICS:
- INTRODUCTION TO PYTHON LISTS
- ACCESSING PYTHON LISTS
- ADDING NEW ELEMENTS IN THE LISTS
- UPDATING PYTHON LISTS
- REMOVING ELEMENT IN THE LISTS
- PYTHON LIST SLICING OPERATION
- USING STEP SIZE IN LIST SLICING OPERATION
- LIST SORTING OPERATIONS
- PYTHON LIST BUILT-IN FUNCTIONS
- REMOVING DUPLICATES USING PYTHON SET OPERATOR
- PYTHON SHALLOW COPY OPERATIONS