INTRODUCTION TO PYTHON TUPLE
INTRODUCTION
- Tuples in python are ordered list of fields
- Fields in tuple are its elements as we saw in lists.
- Tuple are enclosed with rounded brackets
- Tuples can be empty
- Tuples are accessed by index number. Tuple index starts with 0.
- Tuple allows duplicate fields
TUPLE DECLARATION
Tuple can be declared in various ways.
# Tuple declaration example 1
# Integer Tuple declaration
int_tuple = (400, 200, 100, 500)
print("\nFields in the tuple -> ", int_tuple)
# String Tuple declaration
str_tuple = ('Python', 'Java', 'C++')
print("\nFields in the tuple -> ", str_tuple)
# Mixed Tuple declaration
mixed_tuple = (400, 'Python', 5.8, True)
print("\nFields in the tuple -> ", mixed_tuple)
# Nested Mixed Tuple declaration
nested_mixed_tuple = (400, 'Python', 5.8, True, (1, 2, 5), [6, 8, 3])
print("\nFields in the tuple -> ", nested_mixed_tuple)
INTRODUCTION TO PYTHON TUPLE : Output
Fields in the tuple -> (400, 200, 100, 500)
Fields in the tuple -> ('Python', 'Java', 'C++')
Fields in the tuple -> (400, 'Python', 5.8, True)
Fields in the tuple -> (400, 'Python', 5.8, True, (1, 2, 5), [6, 8, 3])
Usually tuple is enclosed by rounded brackets. But if we ignore brackets then also python will accept the declaration by considering the comma separated values. Any comma separated values without brackets will be considered as tuple.
# Tuple declaration example 2
# Integer Tuple declaration
int_tuple = 400, 200, 100, 500
print("\nFields in the tuple -> ", int_tuple)
# String Tuple declaration
str_tuple = 'Python', 'Java', 'C++'
print("\nFields in the tuple -> ", str_tuple)
# Mixed Tuple declaration
mixed_tuple = 400, 'Python', 5.8, True
print("\nFields in the tuple -> ", mixed_tuple)
# Nested Mixed Tuple declaration
nested_mixed_tuple = 400, 'Python', 5.8, True, (1, 2, 5), [6, 8, 3]
print("\nFields in the tuple -> ", nested_mixed_tuple)
INTRODUCTION TO PYTHON TUPLE : Output
Fields in the tuple -> (400, 200, 100, 500)
Fields in the tuple -> ('Python', 'Java', 'C++')
Fields in the tuple -> (400, 'Python', 5.8, True)
Fields in the tuple -> (400, 'Python', 5.8, True, (1, 2, 5), [6, 8, 3])
If we assign multiple variables with the same tuple variable then each field of the tuple will be stored in each respective variable.
# Tuple declaration example 3
# Integer Tuple declaration
int_tuple = (400, 200, 100)
print("\nFields in the tuple -> ", int_tuple)
a, b, c = int_tuple
print("\nValue of a -> ", a)
print("Value of b -> ", b)
print("Value of c -> ", c)
# String Tuple declaration
str_tuple = ('Python', 'Java', 'C++')
print("\nFields in the tuple -> ", str_tuple)
a, b, c = str_tuple
print("\nValue of a -> ", a)
print("Value of b -> ", b)
print("Value of c -> ", c)
# Nested Mixed Tuple declaration
nested_mixed_tuple = (400, 'Python', 5.8, True, (1, 2, 5), [6, 8, 3])
print("\nFields in the tuple -> ", nested_mixed_tuple)
a, b, c, d, e, f = nested_mixed_tuple
print("\nValue of a -> ", a)
print("Value of b -> ", b)
print("Value of c -> ", c)
print("Value of d -> ", d)
print("Value of e -> ", e)
print("Value of f -> ", f)
INTRODUCTION TO PYTHON TUPLE : Output
Fields in the tuple -> (400, 200, 100)
Value of a -> 400
Value of b -> 200
Value of c -> 100
Fields in the tuple -> ('Python', 'Java', 'C++')
Value of a -> Python
Value of b -> Java
Value of c -> C++
Fields in the tuple -> (400, 'Python', 5.8, True, (1, 2, 5), [6, 8, 3])
Value of a -> 400
Value of b -> Python
Value of c -> 5.8
Value of d -> True
Value of e -> (1, 2, 5)
Value of f -> [6, 8, 3]
TUPLE TYPE
INTEGER TUPLE
Integer tuple contains all the integer fields.
# Integer tuple example 1
int_tuple = (400, 200, 600, 100, 150, 500)
print("\nFields in the tuple -> ", int_tuple)
print("\n1st field of tuple int_tuple ->", int_tuple[0])
print("2nd field of tuple int_tuple ->", int_tuple[1])
print("3rd field of tuple int_tuple ->", int_tuple[2])
print("4th field of tuple int_tuple ->", int_tuple[3])
print("5th field of tuple int_tuple ->", int_tuple[4])
print("6th field of tuple int_tuple ->", int_tuple[5])
INTRODUCTION TO PYTHON TUPLE : Output
Fields in the tuple -> (400, 200, 600, 100, 150, 500)
1st field of tuple int_tuple -> 400
2nd field of tuple int_tuple -> 200
3rd field of tuple int_tuple -> 600
4th field of tuple int_tuple -> 100
5th field of tuple int_tuple -> 150
6th field of tuple int_tuple -> 500
If we multiply an integer tuple variable with an integer n then all the tuple fields will be repeated n times inside the tuple.
# Integer tuple example 2
int_tuple = (400, 200, 600, 100, 150, 500)
print("\nFields in the tuple -> ", int_tuple)
print("\n1st field of tuple int_tuple ->", int_tuple[0])
print("2nd field of tuple int_tuple ->", int_tuple[1])
print("3rd field of tuple int_tuple ->", int_tuple[2])
print("4th field of tuple int_tuple ->", int_tuple[3])
print("5th field of tuple int_tuple ->", int_tuple[4])
print("6th field of tuple int_tuple ->", int_tuple[5])
int_tuple = int_tuple * 2
print("\nFields in the tuple -> ", int_tuple)
print("\n1st field of tuple int_tuple ->", int_tuple[0])
print("2nd field of tuple int_tuple ->", int_tuple[1])
print("3rd field of tuple int_tuple ->", int_tuple[2])
print("4th field of tuple int_tuple ->", int_tuple[3])
print("5th field of tuple int_tuple ->", int_tuple[4])
print("6th field of tuple int_tuple ->", int_tuple[5])
print("7th field of tuple int_tuple ->", int_tuple[6])
print("8th field of tuple int_tuple ->", int_tuple[7])
print("9th field of tuple int_tuple ->", int_tuple[8])
print("10th field of tuple int_tuple ->", int_tuple[9])
print("11th field of tuple int_tuple ->", int_tuple[10])
print("12th field of tuple int_tuple ->", int_tuple[11])
INTRODUCTION TO PYTHON TUPLE : Output
Fields in the tuple -> (400, 200, 600, 100, 150, 500)
1st field of tuple int_tuple -> 400
2nd field of tuple int_tuple -> 200
3rd field of tuple int_tuple -> 600
4th field of tuple int_tuple -> 100
5th field of tuple int_tuple -> 150
6th field of tuple int_tuple -> 500
Fields in the tuple -> (400, 200, 600, 100, 150, 500, 400, 200, 600, 100, 150, 500)
1st field of tuple int_tuple -> 400
2nd field of tuple int_tuple -> 200
3rd field of tuple int_tuple -> 600
4th field of tuple int_tuple -> 100
5th field of tuple int_tuple -> 150
6th field of tuple int_tuple -> 500
7th field of tuple int_tuple -> 400
8th field of tuple int_tuple -> 200
9th field of tuple int_tuple -> 600
10th field of tuple int_tuple -> 100
11th field of tuple int_tuple -> 150
12th field of tuple int_tuple -> 500
STRING TUPLE
String tuple contains all the string fields.
# String tuple example 1
str_tuple = ('Python', 'Java', 'C++')
print("\nFields in the tuple -> ", str_tuple)
print("\n1st field of tuple str_tuple ->", str_tuple[0])
print("2nd field of tuple str_tuple ->", str_tuple[1])
print("3rd field of tuple str_tuple ->", str_tuple[2])
INTRODUCTION TO PYTHON TUPLE : Output
If we multiply a string tuple variable with an integer n then all the tuple fields will be repeated n times inside the tuple.
# String tuple example 2
str_tuple = ('Python', 'Java', 'C++')
print("\nFields in the tuple -> ", str_tuple)
print("\n1st field of tuple str_tuple ->", str_tuple[0])
print("2nd field of tuple str_tuple ->", str_tuple[1])
print("3rd field of tuple str_tuple ->", str_tuple[2])
str_tuple = str_tuple * 2
print("\nFields in the tuple -> ", str_tuple)
print("\n1st field of tuple str_tuple ->", str_tuple[0])
print("2nd field of tuple str_tuple ->", str_tuple[1])
print("3rd field of tuple str_tuple ->", str_tuple[2])
print("4th field of tuple str_tuple ->", str_tuple[3])
print("5th field of tuple str_tuple ->", str_tuple[4])
print("6th field of tuple str_tuple ->", str_tuple[5])
INTRODUCTION TO PYTHON TUPLE : Output
Fields in the tuple -> ('Python', 'Java', 'C++')
1st field of tuple str_tuple -> Python
2nd field of tuple str_tuple -> Java
3rd field of tuple str_tuple -> C++
Fields in the tuple -> ('Python', 'Java', 'C++', 'Python', 'Java', 'C++')
1st field of tuple str_tuple -> Python
2nd field of tuple str_tuple -> Java
3rd field of tuple str_tuple -> C++
4th field of tuple str_tuple -> Python
5th field of tuple str_tuple -> Java
6th field of tuple str_tuple -> C++
MIXED TUPLE
Mixed tuple contains fields with multiple data types like integer, string, floating numbers, boolean etc.
# Mixed tuple example 1
mixed_tuple = (400, 'Python', 5.8, True)
print("\nFields in the tuple -> ", mixed_tuple)
print("\n1st field of tuple mixed_tuple ->", mixed_tuple[0])
print("2nd field of tuple mixed_tuple ->", mixed_tuple[1])
print("3rd field of tuple mixed_tuple ->", mixed_tuple[2])
print("4th field of tuple mixed_tuple ->", mixed_tuple[3])
INTRODUCTION TO PYTHON TUPLE : Output
Fields in the tuple -> (400, 'Python', 5.8, True)
1st field of tuple mixed_tuple -> 400
2nd field of tuple mixed_tuple -> Python
3rd field of tuple mixed_tuple -> 5.8
4th field of tuple mixed_tuple -> True
If we multiply a mixed tuple variable with an integer n then all the tuple fields will be repeated n times inside the tuple.
# Mixed tuple example 2
mixed_tuple = (400, 'Python', 5.8, True)
print("\nFields in the tuple -> ", mixed_tuple)
print("\n1st field of tuple mixed_tuple ->", mixed_tuple[0])
print("2nd field of tuple mixed_tuple ->", mixed_tuple[1])
print("3rd field of tuple mixed_tuple ->", mixed_tuple[2])
print("4th field of tuple mixed_tuple ->", mixed_tuple[3])
mixed_tuple = mixed_tuple * 2
print("\nFields in the tuple -> ", mixed_tuple)
print("\n1st field of tuple mixed_tuple ->", mixed_tuple[0])
print("2nd field of tuple mixed_tuple ->", mixed_tuple[1])
print("3rd field of tuple mixed_tuple ->", mixed_tuple[2])
print("4th field of tuple mixed_tuple ->", mixed_tuple[3])
print("5th field of tuple mixed_tuple ->", mixed_tuple[4])
print("6th field of tuple mixed_tuple ->", mixed_tuple[5])
print("7th field of tuple mixed_tuple ->", mixed_tuple[6])
print("8th field of tuple mixed_tuple ->", mixed_tuple[7])
INTRODUCTION TO PYTHON TUPLE : Output
Fields in the tuple -> (400, 'Python', 5.8, True)
1st field of tuple mixed_tuple -> 400
2nd field of tuple mixed_tuple -> Python
3rd field of tuple mixed_tuple -> 5.8
4th field of tuple mixed_tuple -> True
Fields in the tuple -> (400, 'Python', 5.8, True, 400, 'Python', 5.8, True)
1st field of tuple mixed_tuple -> 400
2nd field of tuple mixed_tuple -> Python
3rd field of tuple mixed_tuple -> 5.8
4th field of tuple mixed_tuple -> True
5th field of tuple mixed_tuple -> 400
6th field of tuple mixed_tuple -> Python
7th field of tuple mixed_tuple -> 5.8
8th field of tuple mixed_tuple -> True
NESTED TUPLE
Tuple can contain other complex data type like tuple, list, dictionaries, sets etc.
# Mixed tuple example 3
mixed_tuple = (400, 'Python', 5.8, True, (1, 2, 5), [6, 8, 3])
print("\nFields in the tuple -> ", mixed_tuple)
print("\n1st field of tuple mixed_tuple ->", mixed_tuple[0])
print("2nd field of tuple mixed_tuple ->", mixed_tuple[1])
print("3rd field of tuple mixed_tuple ->", mixed_tuple[2])
print("4th field of tuple mixed_tuple ->", mixed_tuple[3])
print("5th field of tuple mixed_tuple ->", mixed_tuple[4])
print("6th field of tuple mixed_tuple ->", mixed_tuple[5])
INTRODUCTION TO PYTHON TUPLE : Output
Fields in the tuple -> (400, 'Python', 5.8, True, (1, 2, 5), [6, 8, 3])
1st field of tuple mixed_tuple -> 400
2nd field of tuple mixed_tuple -> Python
3rd field of tuple mixed_tuple -> 5.8
4th field of tuple mixed_tuple -> True
5th field of tuple mixed_tuple -> (1, 2, 5)
6th field of tuple mixed_tuple -> [6, 8, 3]
In the above example we can see that the mixed_tuple contains another tuple and a list.
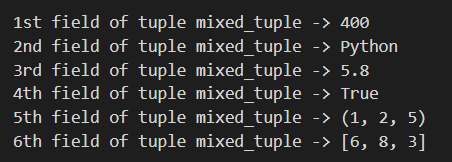
RELATED TOPICS:
- ACCESSING NUMBER TUPLE IN PYTHON
- ACCESSING STRING TUPLE IN PYTHON
- ACCESSING MIXED TUPLE IN PYTHON
- ACCESSING NESTED TUPLE IN PYTHON
- PYTHON TOUPLE SLICING OPERATION
- STEP SIZE IN PYTHON TUPLE SLICING
- IMMUTABILITY OF TUPLE IN PYTHON
- ZIP FUNCTION IN PYTHON TUPLE
- PYTHON TUPLE SORTING OPERATIONS
- PYTHON TUPLE BUILT-IN FUNCTIONS
- REOVING TUPLE DUPLICATES USING SET
- PYTHON TUPLE SHALLOW COPY
- PYTHON TUPLE DEEP COPY
Pingback: ACCESSING NUMBER TUPLE IN PYTHON - Sayantan's Blog On Python Programming
Pingback: ACCESSING STRING TUPLE IN PYTHON - Sayantan's Blog On Python Programming
Pingback: ACCESSING MIXED TUPLE IN PYTHON - Sayantan's Blog On Python Programming
Pingback: ACCESSING NESTED TUPLE IN PYTHON - Sayantan's Blog On Python Programming
Pingback: PYTHON TOUPLE SLICING OPERATION - Sayantan's Blog On Python Programming
Pingback: STEP SIZE IN PYTHON TUPLE SLICING - Sayantan's Blog On Python Programming
Pingback: PYTHON TUPLE BUILT-IN FUNCTIONS - Sayantan's Blog On Python Programming
Pingback: REOVING TUPLE DUPLICATES USING SET - Sayantan's Blog On Python Programming
Pingback: PYTHON TUPLE DEEP COPY - Sayantan's Blog On Python Programming