PYTHON INTEGERS
Types of Number variables:
- INTEGER
- FLOATING POINT NUMBER
WORKING WITH INTEGER VARIABLES:
Simple adding using variables:
# Simple adding using variables example
a = 7
b = 24
print("\nValue of a -> ", a)
print("\nValue of b -> ", b)
print("\nTotal of a and b -> ", (a + b))
PYTHON INTEGERS : Output
Value of a -> 7
Value of b -> 24
Total of a and b -> 31
In the above example we have used two variables a and b. a contains 7 and b contains 24.
We have printed both the variables. Then we have also printed the sum of a and b.
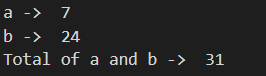
Adding one variable to itself:
# Adding one variable to itself example
a = 7
print("\nValue of a -> ", a)
a += a
print("\nTotal of a += a -> ", a)
PYTHON INTEGERS : Output
Value of a -> 7
Total of a += a -> 14
Here += indicates that a is added a.
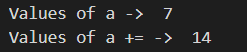
Subtracting one variable to itself:
# Subtracting one variable to itself example
a = 7
print("\nValue of a -> ", a)
a -= a
print("\nTotal of a -= a -> ", a)
PYTHON INTEGERS : Output
Value of a -> 7
Total of a -= a -> 0
Multiplying one variable to itself:
# Multiplying one variable to itself example
a = 7
print("\nValue of a -> ", a)
a *= a
print("\nTotal of a *= a -> ", a)
PYTHON INTEGERS : Output
Value of a -> 7
Total of a *= a -> 49
Dividing one variable to itself:
# Dividing one variable to itself example
a = 7
print("\nValue of a -> ", a)
a /= a
print("\nTotal of a /= a -> ", a)
PYTHON INTEGERS : Output
Value of a -> 7
Total of a /= a -> 1.0
Dividing one Number variable with another Number:
Dividing one number variable with another number results in a decimal number or floating point number.
# Dividing one Number variable with another Number example
a = 7
print("\nValue of a -> ", a)
print("\nData Type of a -> ", type(a))
b = 14
print("\nValue of b -> ", b)
print("\nData Type of b -> ", type(b))
print("\nValue of b / a -> ", b/a)
c = b/a
print("\nValue of c -> ", c)
print("\nData Type of c -> ", type(c))
PYTHON INTEGERS : Output
alue of a -> 7
Data Type of a -> <class 'int'>
Value of b -> 14
Data Type of b -> <class 'int'>
Value of b / a -> 2.0
Value of c -> 2.0
Data Type of c -> <class 'float'>
Calculation using Integer variables:
print("\nMonthly Budget Calculation :->")
salary = 40000
house_rent = 7000
grocery = 5000
travel = 1000
bills = 5000
others = 3000
print("\nSalary -> ", salary)
print("\nHouse Rent -> ", house_rent)
print("\nGrocery -> ", grocery)
print("\nTravel-> ", travel)
print("\nBills -> ", bills)
print("\nOthers -> ", others)
saving = (salary - (house_rent + grocery + travel + bills + others))
print("\nSaving -> ", saving)
In the above example we have created a list of variables which indicate different components of monthly budget.
Finally we have used one variable saving to calculate the balance of all components deducted from salary.
Monthly Budget Calculation :->
Salary -> 40000
House Rent -> 7000
Grocery -> 5000
Travel-> 1000
Bills -> 5000
Others -> 3000
Saving -> 19000
# Calculation using Integer variables example
print("\nCalculation total price of a mobile phone :->")
emi = 4000
no_of_installments = 12
print("\nEMI -> ", emi)
print("\nNumber Of Installments -> ", no_of_installments)
total_price = emi * no_of_installments
print("\nTotal Price -> ", total_price)
In the above example we have calculated the total price of a Mobile Phone taken in installments.
PYTHON INTEGERS : Output
Calculation total price of a mobile phone :->
EMI -> 4000
Number Of Installments -> 12
Total Price -> 48000
Working with String and Integer variables:
# Working with String and Integer variables example
print("\nCalculation total price of a mobile phone :->")
a = "Hello World "
print("\nValue of a -> ", a)
b = 3
print("\nValue of b -> ", b)
print("\nValue of a * b -> ", a * b)
If we multiply a string variable with an integer then the string will be visible integer times. In the above example we have multiplied the string variable b with integer variable a which stores the value of 3. So the string b which holds the value Hello World will be repeated 3 times.
Calculation total price of a mobile phone :->
Value of a -> Hello World
Value of b -> 3
Value of a * b -> Hello World Hello World Hello World
RELATED TOPICS:
Pingback: PYTHON FLOATING POINT NUMBERS - Sayantan's Blog On Python Programming
Pingback: PYTHON CALCULATORS - Sayantan's Blog On Python Programming