STEP SIZE IN PYTHON TUPLE SLICING
Step size allows us to put filter on sliced data.
POSITIVE STEP SIZE IN TUPLE SLICING
Syntax : [start_index : end_index : setp_size]
# Slicing tuple fields with step size example 1
int_tuple = (400, 200, 600, 100, 150, 500)
print("\nFields of original tuple ->", int_tuple)
int_tuple_slice = int_tuple[0:6]
print("\nFields of sliced tuple ->", int_tuple_slice)
int_tuple_slice = int_tuple[0:6:1]
print("\nFields of sliced tuple with step size ->", int_tuple_slice)
STEP SIZE IN PYTHON TUPLE SLICING : Output
Fields of original tuple -> (400, 200, 600, 100, 150, 500)
Fields of sliced tuple -> (400, 200, 600, 100, 150, 500)
Fields of sliced tuple with step size -> (400, 200, 600, 100, 150, 500)
# Slicing tuple fields with step size example 2
int_tuple = (400, 200, 600, 100, 150, 500)
print("\nFields of original tuple ->", int_tuple)
int_tuple_slice = int_tuple[0:6]
print("\nFields of sliced tuple ->", int_tuple_slice)
int_tuple_slice = int_tuple[0:6:2]
print("\nFields of sliced tuple with step size ->", int_tuple_slice)
STEP SIZE IN PYTHON TUPLE SLICING : Output
Fields of original tuple -> (400, 200, 600, 100, 150, 500)
Fields of sliced tuple -> (400, 200, 600, 100, 150, 500)
Fields of sliced tuple with step size -> (400, 600, 150)
In the above example, we have used the complete list in the slicing operation but then have used the step size as 2. Step size 2 indicates that every alternative elements will be returned. That’s why 400, 600, 150 has been returned.
# Slicing tuple fields with step size example 3
int_tuple = (400, 200, 600, 100, 150, 500)
print("\nFields of original tuple ->", int_tuple)
int_tuple_slice = int_tuple[0:4]
print("\nFields of sliced tuple ->", int_tuple_slice)
int_tuple_slice = int_tuple[0:4:2]
print("\nFields of sliced tuple with step size ->", int_tuple_slice)
STEP SIZE IN PYTHON TUPLE SLICING : Output
Fields of original tuple -> (400, 200, 600, 100, 150, 500)
Fields of sliced tuple -> (400, 200, 600, 100)
Fields of sliced tuple with step size -> (400, 600)
# Slicing tuple fields with step size example 4
int_tuple = (400, 200, 600, 100, 150, 500)
print("\nFields of original tuple ->", int_tuple)
int_tuple_slice = int_tuple[2:4]
print("\nFields of sliced tuple ->", int_tuple_slice)
int_tuple_slice = int_tuple[2:4:2]
print("\nFields of sliced tuple with step size ->", int_tuple_slice)
STEP SIZE IN PYTHON TUPLE SLICING : Output
Fields of original tuple -> (400, 200, 600, 100, 150, 500)
Fields of sliced tuple -> (600, 100)
Fields of sliced tuple with step size -> (600,)
# Slicing tuple fields with step size example 5
int_tuple = (400, 200, 600, 100, 150, 500)
print("\nFields of original tuple ->", int_tuple)
int_tuple_slice = int_tuple[0:5]
print("\nFields of sliced tuple ->", int_tuple_slice)
int_tuple_slice = int_tuple[0:5:3]
print("\nFields of sliced tuple with step size ->", int_tuple_slice)
STEP SIZE IN PYTHON TUPLE SLICING : Output
Fields of original tuple -> (400, 200, 600, 100, 150, 500)
Fields of sliced tuple -> (400, 200, 600, 100, 150)
Fields of sliced tuple with step size -> (400, 100)
In the above example, we have sliced the data up to index position 4. Then we have use the step size 3 which has filtered out every 3rd alternative elements of sliced data. That’s why 400 and 100 have been returned.
NEGATIVE STEP SIZE IN TUPLE SLICING
Python allows negative step sizing in tuple.
# Slicing tuple fields with negative step size example 1
int_tuple = (400, 200, 600, 100, 150, 500)
print("\nFields of original tuple ->", int_tuple)
int_tuple_slice = int_tuple[0:]
print("\nFields of sliced tuple ->", int_tuple_slice)
int_tuple_slice = int_tuple[::-1]
print("\nFields of sliced tuple with step size ->", int_tuple_slice)
STEP SIZE IN PYTHON TUPLE SLICING : Output
Fields of original tuple -> (400, 200, 600, 100, 150, 500)
Fields of sliced tuple -> (400, 200, 600, 100, 150, 500)
Fields of sliced tuple with step size -> (500, 150, 100, 600, 200, 400)
In the above example, we have used negative step size as -1. It will sorted the tuple in reverse order.
# Slicing tuple fields with negative step size example 2
int_tuple = (400, 200, 600, 100, 150, 500)
print("\nFields of original tuple ->", int_tuple)
int_tuple_slice = int_tuple[0:]
print("\nFields of sliced tuple ->", int_tuple_slice)
int_tuple_slice = int_tuple[::-2]
print("\nFields of sliced tuple with step size ->", int_tuple_slice)
STEP SIZE IN PYTHON TUPLE SLICING : Output
Fields of original tuple -> (400, 200, 600, 100, 150, 500)
Fields of sliced tuple -> (400, 200, 600, 100, 150, 500)
Fields of sliced tuple with step size -> (500, 100, 200)
Till now we have used the entire tuple for step size operation. Now we will use the shorter range in tuple.
In order to use the negative step size we have to use the higher start index and lower end index. Otherwise, python will return empty tuple.
# Slicing tuple fields with negative step size example 3
int_tuple = (400, 200, 600, 100, 150, 500)
print("\nFields of original tuple ->", int_tuple)
int_tuple_slice = int_tuple[0:3:-2]
print("\nFields of sliced tuple with step size ->", int_tuple_slice)
STEP SIZE IN PYTHON TUPLE SLICING : Output
Fields of original tuple -> (400, 200, 600, 100, 150, 500)
Fields of sliced tuple with step size -> ()
In the above example, we have used lower start index and higher end index, that’s why python has returned empty tuple. Because, python can not go from 0 to 3 in reverse order. It can go from 3 to 0 in reverse order as we can view in the next example.
# Slicing tuple fields with negative step size example 4
int_tuple = (400, 200, 600, 100, 150, 500)
print("\nFields of original tuple ->", int_tuple)
int_tuple_slice = int_tuple[3:0:-2]
print("\nFields of sliced tuple with step size ->", int_tuple_slice)
STEP SIZE IN PYTHON TUPLE SLICING : Output
Fields of original tuple -> (400, 200, 600, 100, 150, 500)
Fields of sliced tuple with step size -> (100, 200)
In the above example, the start index is 3 which indicates the field 100. End index is 0 (exclusive) which indicates the field 200. Now, step size -2 will return every 2nd alternative field starting from 100 in reverse order. That’s why 100 and 200 has been returned by python.
# Slicing tuple fields with negative step size example 5
int_tuple = (400, 200, 600, 100, 150, 500)
print("\nFields of original tuple ->", int_tuple)
int_tuple_slice = int_tuple[-4:0:-1]
print("\nFields of sliced tuple with step size ->", int_tuple_slice)
STEP SIZE IN PYTHON TUPLE SLICING : Output
Fields of original tuple -> (400, 200, 600, 100, 150, 500)
Fields of sliced tuple with step size -> (600, 200)
In the above example, we have seen that starting index is -4 which indicates the field 600 and end index is 0 (exclusive) which indicates the field 200. Now, step size is -1. So, every field will be listed starting from 600 to 200. That’s why 600 and 200 has been returned by python.
USING SLICING OPERATION TO CREATE NEW TUPLE FIELDS
We can not insert new fields in a tuple because ‘tuple’ object does not support item assignment.
# Adding new tuple fields with Slicing operation example 1
int_tuple = (400, 200, 600, 100, 150, 500)
print("\nFields of original tuple ->", int_tuple)
int_tuple[6:0] = (750, 850)
print("\nRevised fields of original tuple ->", int_tuple)
STEP SIZE IN PYTHON TUPLE SLICING : Output
Fields of original tuple -> (400, 200, 600, 100, 150, 500)
Traceback (most recent call last):
File "d:\PYTHON\PROGRAM\tempCodeRunnerFile.python", line 6, in <module>
int_tuple[6:0] = (750, 850)
~~~~~~~~~^^^^^
TypeError: 'tuple' object does not support item assignment
In List object we have seen that we can create new elements by using above assignment operation. But in tuple its not allowed.
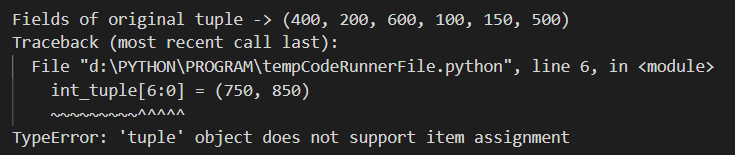
RELATED TOPICS:
- INTRODUCTION TO PYTHON TUPLE
- ACCESSING NUMBER TUPLE IN PYTHON
- ACCESSING STRING TUPLE IN PYTHON
- ACCESSING MIXED TUPLE IN PYTHON
- ACCESSING NESTED TUPLE IN PYTHON
- PYTHON TOUPLE SLICING OPERATION
- IMMUTABILITY OF TUPLE IN PYTHON
- ZIP FUNCTION IN PYTHON TUPLE
- PYTHON TUPLE SORTING OPERATIONS
- PYTHON TUPLE BUILT-IN FUNCTIONS
- REOVING TUPLE DUPLICATES USING SET
- PYTHON TUPLE SHALLOW COPY
- PYTHON TUPLE DEEP COPY