UPDATING PYTHON LISTS
INTRODUCTIONS
UPDATING INTEGER LISTS
To update an element in the list we will assign a new element in a given index position.
# Updating List elements example 1
int_list = [400, 200, 600, 100, 150, 200]
print("\nElement of list int_list at index 0->", int_list[0])
print("Element of list int_list at index 1->", int_list[1])
print("Element of list int_list at index 2->", int_list[2])
print("Element of list int_list at index 3->", int_list[3])
print("Element of list int_list at index 4->", int_list[4])
print("Element of list int_list at index 5->", int_list[5])
print("\nNumber of element in the list int_list ->", len(int_list))
int_list[2] = 300
int_list[5] = 120
print("\nElement of list int_list at index 0->", int_list[0])
print("Element of list int_list at index 1->", int_list[1])
print("Element of list int_list at index 2->", int_list[2])
print("Element of list int_list at index 3->", int_list[3])
print("Element of list int_list at index 4->", int_list[4])
print("Element of list int_list at index 5->", int_list[5])
print("\nRevised Number of element in the list int_list ->", len(int_list))
UPDATING PYTHON LISTS : Output
Element of list int_list at index 0-> 400
Element of list int_list at index 1-> 200
Element of list int_list at index 2-> 600
Element of list int_list at index 3-> 100
Element of list int_list at index 4-> 150
Element of list int_list at index 5-> 200
Number of element in the list int_list -> 6
Element of list int_list at index 0-> 400
Element of list int_list at index 1-> 200
Element of list int_list at index 2-> 300
Element of list int_list at index 3-> 100
Element of list int_list at index 4-> 150
Element of list int_list at index 5-> 120
Revised Number of element in the list int_list -> 6
UPDATING STRING LISTS
In the above example, we have updated the list at index position 2 and 5. Earlier 600 was at index position 2 which is replaced by 300 and at index position 5, 200 is replaced by 120.
# Updating List elements example 2
str_list = ['Python', 'Java', 'C++', 'C', 'PHP', 'XML']
print("\nElement of list str_list at index 0->", str_list[0])
print("Element of list str_list at index 1->", str_list[1])
print("Element of list str_list at index 2->", str_list[2])
print("Element of list str_list at index 3->", str_list[3])
print("Element of list str_list at index 4->", str_list[4])
print("Element of list str_list at index 5->", str_list[5])
print("\nNumber of element in the list str_list ->", len(str_list))
str_list[2] = 'Word'
str_list[5] = 'Excel'
print("\nElement of list str_list at index 0->", str_list[0])
print("Element of list str_list at index 1->", str_list[1])
print("Element of list str_list at index 2->", str_list[2])
print("Element of list str_list at index 3->", str_list[3])
print("Element of list str_list at index 4->", str_list[4])
print("Element of list str_list at index 5->", str_list[5])
print("\nRevised Number of element in the list str_list ->", len(str_list))
UPDATING PYTHON LISTS : Output
Element of list str_list at index 0-> Python
Element of list str_list at index 1-> Java
Element of list str_list at index 2-> C++
Element of list str_list at index 3-> C
Element of list str_list at index 4-> PHP
Element of list str_list at index 5-> XML
Number of element in the list str_list -> 6
Element of list str_list at index 0-> Python
Element of list str_list at index 1-> Java
Element of list str_list at index 2-> Word
Element of list str_list at index 3-> C
Element of list str_list at index 4-> PHP
Element of list str_list at index 5-> Excel
Revised Number of element in the list str_list -> 6
In the above example, we have updated the list at index position 2 and 5. Earlier C++ was at index position 2 which is replaced by Word and at index position 5, XML is replaced by Excel.
UPDATING NESTED LISTS
UPDATING INTEGER NESTED LISTS
We can update the element of a list which is an element of another bigger list.
# Updating Nested List elements example 1
int_list = [400, 200, 600, [10, 20, 30], 150, 200]
print("\nElement of list int_list at index 0->", int_list[0])
print("Element of list int_list at index 1->", int_list[1])
print("Element of list int_list at index 2->", int_list[2])
print("Element of list int_list at index [3][0]->", int_list[3][0])
print("Element of list int_list at index [3][1]->", int_list[3][1])
print("Element of list int_list at index [3][2]->", int_list[3][2])
print("Element of list int_list at index 4->", int_list[4])
print("Element of list int_list at index 5->", int_list[5])
print("\nNumber of element in the list int_list ->", len(int_list))
int_list[3][0] = 40
int_list[3][2] = 50
print("\nElement of list int_list at index 0->", int_list[0])
print("Element of list int_list at index 1->", int_list[1])
print("Element of list int_list at index 2->", int_list[2])
print("Element of list int_list at index [3][0]->", int_list[3][0])
print("Element of list int_list at index [3][1]->", int_list[3][1])
print("Element of list int_list at index [3][2]->", int_list[3][2])
print("Element of list int_list at index 4->", int_list[4])
print("Element of list int_list at index 5->", int_list[5])
print("\nNumber of element in the list int_list ->", len(int_list))
UPDATING PYTHON LISTS : Output
Element of list int_list at index 0-> 400
Element of list int_list at index 1-> 200
Element of list int_list at index 2-> 600
Element of list int_list at index [3][0]-> 10
Element of list int_list at index [3][1]-> 20
Element of list int_list at index [3][2]-> 30
Element of list int_list at index 4-> 150
Element of list int_list at index 5-> 200
Number of element in the list int_list -> 6
Element of list int_list at index 0-> 400
Element of list int_list at index 1-> 200
Element of list int_list at index 2-> 600
Element of list int_list at index [3][0]-> 40
Element of list int_list at index [3][1]-> 20
Element of list int_list at index [3][2]-> 50
Element of list int_list at index 4-> 150
Element of list int_list at index 5-> 200
Number of element in the list int_list -> 6
In the above example, we have taken a list [10, 20, 30] inside the main list int_list and updated the elements at index position 0 and 2. Earlier 10 was at position [3][0] which is replaced by 40. Similarly, 30 was at index position [3][2] which is replaced by 50.
UPDATING STRING NESTED LISTS
# Updating Nested List elements example 2
str_list = ['Python', 'Java', 'C++', ['VB', 'VB.Net', 'VC++'], 'PHP', 'XML']
print("\nElement of list str_list at index 0->", str_list[0])
print("Element of list str_list at index 1->", str_list[1])
print("Element of list str_list at index 2->", str_list[2])
print("Element of list str_list at index [3][0]->", str_list[3][0])
print("Element of list str_list at index [3][1]->", str_list[3][1])
print("Element of list str_list at index [3][2]->", str_list[3][2])
print("Element of list str_list at index 4->", str_list[4])
print("Element of list str_list at index 5->", str_list[5])
print("\nNumber of element in the list str_list ->", len(str_list))
str_list[3][0] = 'Word'
str_list[3][2] = 'Excel'
print("\nElement of list str_list at index 0->", str_list[0])
print("Element of list str_list at index 1->", str_list[1])
print("Element of list str_list at index 2->", str_list[2])
print("Element of list str_list at index [3][0]->", str_list[3][0])
print("Element of list str_list at index [3][1]->", str_list[3][1])
print("Element of list str_list at index [3][2]->", str_list[3][2])
print("Element of list str_list at index 4->", str_list[4])
print("Element of list str_list at index 5->", str_list[5])
print("\nNumber of element in the list str_list ->", len(str_list))
UPDATING PYTHON LISTS : Output
Element of list str_list at index 0-> Python
Element of list str_list at index 1-> Java
Element of list str_list at index 2-> C++
Element of list str_list at index [3][0]-> VB
Element of list str_list at index [3][1]-> VB.Net
Element of list str_list at index [3][2]-> VC++
Element of list str_list at index 4-> PHP
Element of list str_list at index 5-> XML
Number of element in the list str_list -> 6
Element of list str_list at index 0-> Python
Element of list str_list at index 1-> Java
Element of list str_list at index 2-> C++
Element of list str_list at index [3][0]-> Word
Element of list str_list at index [3][1]-> VB.Net
Element of list str_list at index [3][2]-> Excel
Element of list str_list at index 4-> PHP
Element of list str_list at index 5-> XML
Number of element in the list str_list -> 6
In the above example, we have taken a list [‘VB’, ‘VB.Net’, ‘VC++’] inside the main list str_list and updated the elements at index position 0 and 2. Earlier ‘VB’ was at position [3][0] which is replaced by Word. Similarly, ‘VC++’ was at index position [3][2] which is replaced by Excel.
UPDATING A LIST ELEMENT WHICH DOES NOT EXISTS
If we try to update a list element which is not exists then Python will throw “index out of range exception“
# Updating Python Lists where which does not exists example 1
int_list = [400, 200, 600, 100, 150, 200]
print("\nElement of list int_list at index 0->", int_list[0])
print("Element of list int_list at index 1->", int_list[1])
print("Element of list int_list at index 2->", int_list[2])
print("Element of list int_list at index 3->", int_list[3])
print("Element of list int_list at index 4->", int_list[4])
print("Element of list int_list at index 5->", int_list[5])
print("\nNumber of element in the list int_list ->", len(int_list))
int_list[6] = 300
print("\nElement of list int_list at index 0->", int_list[6])
UPDATING PYTHON LISTS : Output
Element of list int_list at index 0-> 400
Element of list int_list at index 1-> 200
Element of list int_list at index 2-> 600
Element of list int_list at index 3-> 100
Element of list int_list at index 4-> 150
Element of list int_list at index 5-> 200
Traceback (most recent call last):
File "d:\PYTHON\PROGRAM\tempCodeRunnerFile.python", line 15, in <module>
int_list[6] = 300
~~~~~~~~^^^
IndexError: list assignment index out of range
Number of element in the list int_list -> 6
# Updating Python Lists where elements which does not exists example 2
str_list = ['Python', 'Java', 'C++', 'VB', 'PHP', 'XML']
print("\nElement of list str_list at index 0->", str_list[0])
print("Element of list str_list at index 1->", str_list[1])
print("Element of list str_list at index 2->", str_list[2])
print("Element of list str_list at index [3][0]->", str_list[3])
print("Element of list str_list at index 4->", str_list[4])
print("Element of list str_list at index 5->", str_list[5])
print("\nNumber of element in the list str_list ->", len(str_list))
str_list[6] = 'Word'
print("\nElement of list str_list at index 0->", str_list[6])
UPDATING PYTHON LISTS : Output
Element of list str_list at index 0-> Python
Element of list str_list at index 1-> Java
Element of list str_list at index 2-> C++
Element of list str_list at index [3][0]-> VB
Element of list str_list at index 4-> PHP
Element of list str_list at index 5-> XML
Number of element in the list str_list -> 6
Traceback (most recent call last):
File "d:\PYTHON\PROGRAM\tempCodeRunnerFile.python", line 12, in <module>
str_list[6] = 'Word'
~~~~~~~~^^^
IndexError: list assignment index out of range
In the above example, we have tried to assign a value at the index position 6 which is not exists. That’s why Python has raised the error.
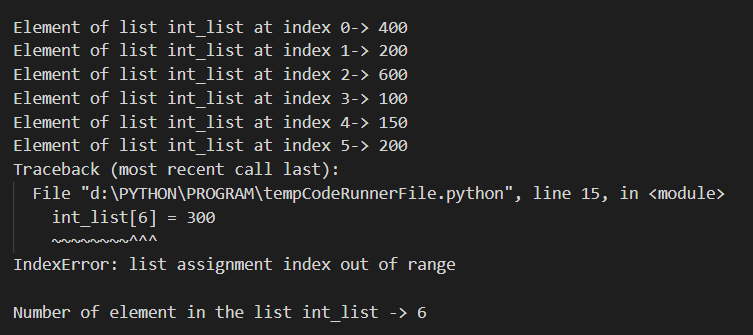
RELATED TOPICS:
- INTRODUCTION TO PYTHON LISTS
- ACCESSING PYTHON LISTS
- ADDING NEW ELEMENTS IN THE LISTS
- REMOVING ELEMENT IN THE LISTS
- PYTHON LIST SLICING OPERATION
- USING STEP SIZE IN LIST SLICING OPERATION
- LIST SORTING OPERATIONS
- PYTHON LIST BUILT-IN FUNCTIONS
- REMOVING DUPLICATES USING PYTHON SET OPERATOR
- PYTHON SHALLOW COPY OPERATIONS
- PYTHON LIST DEEP COPY
Pingback: PYTHON LIST SLICING OPERATION - Sayantan's Blog On Python Programming